标签:
以下程序主要是使用QQ地图对经纬进行逆解析.
1 先写 类程序
using System; using System.Text; using System.IO; using System.Net; using System.Web; using Newtonsoft.Json; using System.Data.SqlTypes; using Microsoft.SqlServer.Server; public static class MSPetShop4 { // latitude; 纬度,浮点数,范围为90 ~ -90 // longitude; 经度,浮点数,范围为180 ~ -180。 [SqlFunction()] public static SqlString GetAdd(string longitude, string latitude) { string url = "http://apis.map.qq.com/ws/geocoder/v1?"; url = url + string.Format("location={0},{1}&coord_type=1&key={2}", latitude, longitude, "XXXXX-XXXXX-XXXXX-XXXXX-XXXXX-XXXXX"); string json = HttpGet(url); Geocoder addrObj = JsonConvert.DeserializeObject<Geocoder>(json); string address = addrObj.result.address; return address; } public static string HttpGet(string url) { HttpWebRequest request = (HttpWebRequest)HttpWebRequest.Create(url); request.Method = "GET"; //request.ContentType = "application/x-www-form-urlencoded"; request.Accept = "*/*"; request.Timeout = 15000; request.AllowAutoRedirect = false; WebResponse response = null; string responseStr = null; try { response = request.GetResponse(); if (response != null) { StreamReader reader = new StreamReader(response.GetResponseStream(), Encoding.UTF8); responseStr = reader.ReadToEnd(); reader.Close(); } } catch (Exception) { throw; } finally { request = null; response = null; } return responseStr; } } //通过QQ地图逆解析到的json 架构的 Modle public class Geocoder { public string status { get; set; } public string message { get; set; } public Result result { get; set; } } public class Street { public string title { get; set; } public Location location { get; set; } public string _distance { get; set; } public string _dir_desc { get; set; } } public class Location { public string lat { get; set; } public string lng { get; set; } } public class Formatted_addresses { public string recommend { get; set; } public string rough { get; set; } } public class Address_component { public string nation { get; set; } public string province { get; set; } public string city { get; set; } public string district { get; set; } public string street { get; set; } public string street_number { get; set; } } public class Ad_info { public string adcode { get; set; } public string name { get; set; } public Location location { get; set; } public string nation { get; set; } public string province { get; set; } public string city { get; set; } public string district { get; set; } } public class Address_reference { public Street crossroad { get; set; } public Street street { get; set; } public Street famous_area { get; set; } public Street town { get; set; } public Street street_number { get; set; } public Street landmark_l1 { get; set; } public Street landmark_l2 { get; set; } } public class Pois { public string id { get; set; } public string title { get; set; } public string address { get; set; } public string category { get; set; } public Location location { get; set; } public string _distance { get; set; } public string _dir_desc { get; set; } } public class Result { public Location location { get; set; } public string address { get; set; } public Formatted_addresses formatted_addresses { get; set; } public Address_component address_component { get; set; } public Ad_info ad_info { get; set; } public Address_reference address_reference { get; set; } public Pois[] pois { get; set; } } //以下为事例: //http://apis.map.qq.com/ws/geocoder/v1/?location=39.984154,116.307490&key=XXXXX-XXXXX-XXXXX-XXXXX-XXXXX-XXXXX&get_poi=1 //{ // "status": 0, // "message": "query ok", // "result": { // "location": { // "lat": 39.984154, // "lng": 116.30749 // }, // "address": "北京市海淀区彩和坊路北四环西路66号", // "formatted_addresses": { // "recommend": "海淀区中关村中国技术交易大厦(彩和坊路西)", // "rough": "海淀区中关村海淀桥东南" // }, // "address_component": { // "nation": "中国", // "province": "北京市", // "city": "北京市", // "district": "海淀区", // "street": "彩和坊路", // "street_number": "北四环西路66号" // }, // "ad_info": { // "adcode": "110108", // "name": "中国,北京市,北京市,海淀区", // "location": { // "lat": 39.984154, // "lng": 116.307487 // }, // "nation": "中国", // "province": "北京市", // "city": "北京市", // "district": "海淀区" // }, // "address_reference": { // "crossroad": { // "title": "彩和坊路/北四环西路辅路(路口)", // "location": { // "lat": 39.985001, // "lng": 116.308113 // }, // "_distance": 104.2, // "_dir_desc": "西南" // }, // "street": { // "title": "彩和坊路", // "location": { // "lat": 39.984154, // "lng": 116.308098 // }, // "_distance": 49.1, // "_dir_desc": "西" // }, // "famous_area": { // "title": "中关村", // "location": { // "lat": 39.984119, // "lng": 116.307503 // }, // "_distance": 0, // "_dir_desc": "内" // }, // "town": { // "title": "海淀街道", // "location": { // "lat": 39.984154, // "lng": 116.307487 // }, // "_distance": 0, // "_dir_desc": "内" // }, // "street_number": { // "title": "北四环西路66号", // "location": { // "lat": 39.984119, // "lng": 116.307503 // }, // "_distance": 0, // "_dir_desc": "" // }, // "landmark_l1": { // "title": "海淀桥", // "location": { // "lat": 39.98513, // "lng": 116.305389 // }, // "_distance": 209.4, // "_dir_desc": "东南" // }, // "landmark_l2": { // "title": "中国技术交易大厦B座", // "location": { // "lat": 39.984112, // "lng": 116.307587 // }, // "_distance": 9.7, // "_dir_desc": "" // } // }, // "pois": [ // { // "id": "12925244666643621769", // "title": "中国技术交易大厦B座", // "address": "北京市海淀区北四环西路66号", // "category": "商务楼宇", // "location": { // "lat": 39.984112, // "lng": 116.307587 // }, // "_distance": 9.7, // "_dir_desc": "" // }, // { // "id": "7586906308739894477", // "title": "海淀桥", // "address": "北京市海淀区", // "category": "地名地址;交通地名", // "location": { // "lat": 39.98513, // "lng": 116.305389 // }, // "_distance": 209.4, // "_dir_desc": "东南" // }, // { // "id": "3629720141162880123", // "title": "中国技术交易大厦", // "address": "北四环西路66号", // "category": "商务楼宇", // "location": { // "lat": 39.984154, // "lng": 116.307487 // }, // "_distance": 0, // "_dir_desc": "内" // }, // { // "id": "2845372667492951071", // "title": "中国技术交易大厦A座", // "address": "北京市海淀区北四环西路66号中国技术交易大厦", // "category": "商务楼宇", // "location": { // "lat": 39.984112, // "lng": 116.307587 // }, // "_distance": 9.7, // "_dir_desc": "" // }, // { // "id": "11208756264581915123", // "title": "第三极创意天地", // "address": "北京市海淀区北四环西路66号", // "category": "商务楼宇", // "location": { // "lat": 39.984058, // "lng": 116.307701 // }, // "_distance": 21.1, // "_dir_desc": "" // }, // { // "id": "3187032738687555052", // "title": "北京中关村创业大街", // "address": "海淀西大街", // "category": "商业步行街", // "location": { // "lat": 39.984055, // "lng": 116.306992 // }, // "_distance": 43.9, // "_dir_desc": "东" // }, // { // "id": "16543580623658120230", // "title": "籍海楼", // "address": "北京市海淀区海淀大街35号", // "category": "商务楼宇", // "location": { // "lat": 39.983677, // "lng": 116.307007 // }, // "_distance": 67.1, // "_dir_desc": "东北" // }, // { // "id": "7246616758286733108", // "title": "基督教堂(彩和坊路)", // "address": "彩和坊路9号", // "category": "教堂", // "location": { // "lat": 39.983391, // "lng": 116.307594 // }, // "_distance": 85.4, // "_dir_desc": "北" // }, // { // "id": "12349575918052623139", // "title": "中国化工大厦", // "address": "北京市海淀区北四环西路62号", // "category": "商务楼宇", // "location": { // "lat": 39.984169, // "lng": 116.308502 // }, // "_distance": 86.5, // "_dir_desc": "西" // }, // { // "id": "13496299173191724318", // "title": "泰鹏大厦", // "address": "北京市海淀区北二街10号", // "category": "商务楼宇", // "location": { // "lat": 39.98378, // "lng": 116.308586 // }, // "_distance": 102.5, // "_dir_desc": "西北" // } // ] // } //}
1 static 静态函数
2 函数需要 SqlFunction() 属性 (需要引用 Microsoft.SqlServer.Server 命名空间)
2 生成DLL文件
这个沒啥好说的,直接生成DLL文件就行.
3 设置数据库
sp_configure ‘clr enabled‘, 1; GO reconfigure GO --查看数据库兼容级别 ,如果只是兼容到100有以上,则要设置为 90 sp_dbcmptlevel --设置兼容级别 sp_dbcmptlevel ‘DATABASE_NAME‘, 90
ALTER DATABASE MSPetShop4 SET TRUSTWORTHY ON
4 数据库中新建程序集
DATABASE_NAME-->可编程性-->程序集 右键-->新建程序集-->
5 数据库中创建函数
CREATE FUNCTION [dbo].GetAddressByLonLat(@longitude nvarchar(max),@latitude nvarchar(max)) RETURNS nvarchar(max) EXTERNAL NAME GetAddresses.MSPetShop4.GetAdd GO
GetAddresses DLL文件名
MSPetShop4 类名
GetAdd 方法名
6 结果
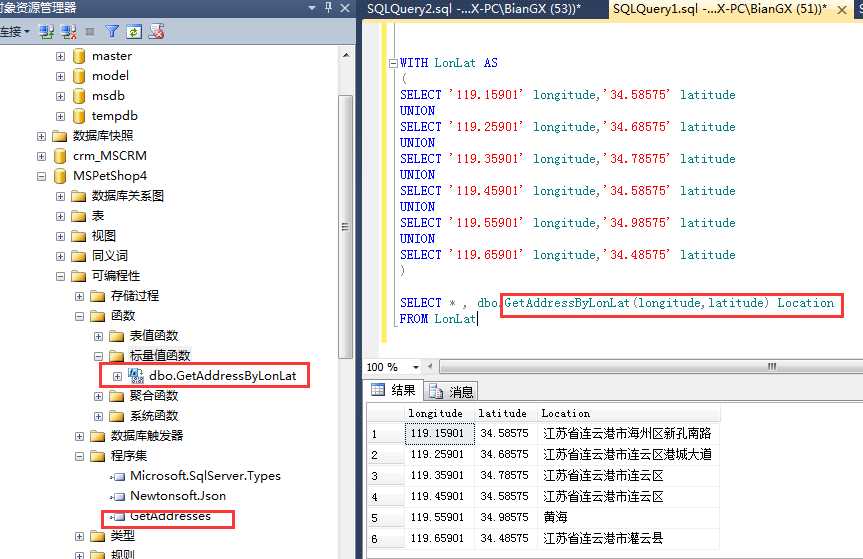
标签:
原文地址:http://www.cnblogs.com/BinBinGo/p/5037867.html