标签:
通讯录管理系统,数据库关系模式为:账户(账户名,登录密码,头像),联系人(ID,姓名,电话,QQ,Email)。主要功能包括:注册,登录,注销账号,修改账户名以及密码,更换头像,以及对联系人的增删改查。
工具:vs2015,sql server2014
数据库关系表:
Account: Contact:
VS中主要界面及代码:
登录主界面:Login
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; namespace Contact_Manage { /// <summary> /// 登录 /// </summary> public partial class Login : Form { //存取用户登录名 public static String txtLogin_Username; public Login() { InitializeComponent(); } //登录 private void btt_Login_Click(object sender, EventArgs e) { //获取用户输入的用户名和密码 txtLogin_Username = tB_Account_Username.Text; String password = tB_Account_Password.Text; if (txtLogin_Username == "" || password == "") { MessageBox.Show("请输入用户名和密码!"); return; } //连接数据库进行数据判断 string sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888"; String sqlcom = " select * from Account where Account_Username=‘" + txtLogin_Username + "‘ and Account_password=‘" + password + "‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); SqlDataReader reader = com.ExecuteReader(); if (reader.Read()) { //打开菜单页面 Menu menu = new Menu(); menu.Show(); } else { MessageBox.Show("用户名或密码错误,请重新输入!"); } con.Close(); //清空文本框 foreach (Control item in this.Controls) { if (item is TextBox) { item.Text = ""; } } } //注册账号事件 private void lb_Regist_Click(object sender, EventArgs e) { Regist rigist = new Regist(); rigist.Show(); } //注销账户事件 private void lb_Logout_Click(object sender, EventArgs e) { //跳出注销界面,验证输入的用户名及密码是否正确 Logout logout = new Logout(); logout.Show(); } //点击密码文本框时将登录头像显示在pictureBox控件中 private void tB_Account_Password_MouseClick(object sender, MouseEventArgs e) { //获取输入的用户名 String username = tB_Account_Username.Text; if (username != "") { //连接数据库,如果该用户名存在则将头像显示 string sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888"; String sqlcom = " select * from Account where Account_Username=‘" + username + "‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); SqlDataReader reader = com.ExecuteReader(); if (reader.Read()) { reader.Close(); //连接数据库,获取该用户名的头像保存路径 //连接数据库 string sqlcon2 = "server=.;database=phone_Book;uid=sa;pwd=666888"; String sqlcom2 = "select Account_Image from Account where Account_Username=‘" + username + "‘"; SqlConnection con2 = new SqlConnection(sqlcon2); SqlCommand com2 = new SqlCommand(sqlcom2, con2); con2.Open(); //返回结果集的第一行第一列 object obj = com2.ExecuteScalar(); //将数据库中读取到的路径放入变量中 String image_Path = obj.ToString(); con2.Close(); pctrB_Image.Image = Image.FromFile(image_Path); //将图片居中显示 pctrB_Image.SizeMode = PictureBoxSizeMode.StretchImage; } else { MessageBox.Show("用户名不存在,请重新输入!"); } con.Close(); } } } }
注册窗体(登录界面中点击注册事件时将跳转到此窗体):Regist
此处更换头像利用了openFileDialog控件打开系统文件会话框,选择图片之后显示在pictureBox控件中,数据库中存放了图片所在的路径,图片的路径可以利用openFileDialog对象.FileName.ToString()获得,点击注册按钮将所有注册信息加入数据库中。
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; namespace Contact_Manage { public partial class Regist : Form { //默认头像路径 public static String imagePath = "F:/VS设计/Contact_Manage/images/1.jpeg"; public Regist() { InitializeComponent(); } private void btt_Regist_Click(object sender, EventArgs e) { //获取用户注册输入的用户名和密码 String regist_Username = tB_Regist_Username.Text; String regist_Password1 = tB_Regist_Password1.Text; string regist_Password2 = tB_Regist_Password2.Text; //判断是否输入了用户名和密码 if (regist_Username == "" || regist_Password1 == "" || regist_Password2 == "") { MessageBox.Show("请输入用户名和密码!"); return; } //判断两次输入的密码是否一致 if (!regist_Password1.Equals(regist_Password2)) { MessageBox.Show("两次输入的密码不一致,请重新输入!"); return; } //连接数据库,判断用户名是否已存在 string sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888"; String sqlcom = " select * from Account where Account_Username=‘" + regist_Username + "‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); SqlDataReader reader = com.ExecuteReader(); if (reader.Read()) { MessageBox.Show("用户名已存在,请重新输入用户名!"); return; } reader.Close(); //验证通过,将用户输入的数据添加进入数据库 String sqlcom2 = " insert into Account(Account_Username,Account_Password,Account_Image) values(‘" + regist_Username + "‘,‘" + regist_Password1 + "‘,‘"+imagePath+"‘)"; SqlCommand com2 = new SqlCommand(sqlcom2, con); int eq=com2.ExecuteNonQuery(); if(eq!=0) { MessageBox.Show("注册成功!将跳转到登录页面!"); this.Close(); } con.Close(); } //当点击用户名文本框时给pictureBox中放置一张默认的图片 private void tB_Regist_Username_MouseClick(object sender, MouseEventArgs e) { //如果pictureBox为空时显示默认图片 if (pctrB_Regist_Image.Image == null) { String imagePath = "F:/VS设计/Contact_Manage/images/1.jpeg"; pctrB_Regist_Image.Image = Image.FromFile(imagePath); pctrB_Regist_Image.SizeMode = PictureBoxSizeMode.StretchImage; } } private void btt_ChangeImage_Click(object sender, EventArgs e) { //自定义OpenFileDialog打开Windows文件对话框选择文件,并保存路径 OpenFileDialog f = new OpenFileDialog(); f.InitialDirectory = Application.StartupPath + @"\images"; f.Filter = "All files (*.*)|*.*|txt files (*.txt)|*.txt"; f.FilterIndex = 1; f.RestoreDirectory = true; if (f.ShowDialog() == DialogResult.OK) { imagePath = f.FileName.ToString(); //将更换的图片显示在注册页面 pctrB_Regist_Image.Image = Image.FromFile(imagePath); //将图片居中显示 pctrB_Regist_Image.SizeMode = PictureBoxSizeMode.StretchImage; } return; } } }
注销账号(在登录界面的注销事件中实例化此窗体):Logout
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; namespace Contact_Manage { /// <summary> /// 注销用户界面 /// </summary> public partial class Logout : Form { //注销账号名 public static string txtLogoutName; public Logout() { InitializeComponent(); } private void btt_Logout_Click(object sender, EventArgs e) { //获取用户输入的将要注销的用户名及密码 String logout_Username = tB_Logout_Username.Text; String logout_Password = tB_Logout_Password.Text; //判断是否输入了用户名及密码 if (logout_Username == "" || logout_Password == "") { MessageBox.Show("请输入要注销的用户名和密码!"); return; } //连接数据库进行数据验证 String sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888;"; String sqlcom = "select * from Account where Account_Username=‘"+logout_Username+"‘ and Account_Password=‘"+logout_Password+"‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom,con); con.Open(); SqlDataReader reader= com.ExecuteReader(); if (reader.Read()) { //如果密码以及用户名正确,提示用户是否确认注销,确定之后将该用户从数据库中删除 txtLogoutName= tB_Logout_Username.Text; Affirm affirm = new Affirm(); affirm.Show(); } else { MessageBox.Show("你输入的用户名或密码不正确,请重新输入!"); } //清空所有文本框 foreach (Control item in this.Controls) { if (item is TextBox) { item.Text = ""; } } con.Close(); } } }
菜单:Menu
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace Contact_Manage { public partial class Menu : Form { public Menu() { InitializeComponent(); } private void btt_Add_Contact_Click(object sender, EventArgs e) { AddContact add = new AddContact(); add.Show(); } private void btt_Manage_Contact_Click(object sender, EventArgs e) { Contact_Manage query= new Contact_Manage(); query.Show(); } //修改密码 private void btt_Modification_Password_Click(object sender, EventArgs e) { Modification_Password modif_Pwd = new Modification_Password(); modif_Pwd.Show(); } //更换头像 private void btt_Change_Image_Click(object sender, EventArgs e) { Change_Image cImg = new Change_Image(); cImg.Show(); } //修改账户名 private void btt_Mdfct_Username_Click(object sender, EventArgs e) { Mdfct_Acct_Username m = new Mdfct_Acct_Username(); m.Show(); } } }
添加联系人:AddContact
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; namespace Contact_Manage { /// <summary> /// 添加联系人 /// </summary> public partial class AddContact : Form { public AddContact() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { //判断是否有必填项目未输入的情况 if (tB_Name.Text.Length == 0 && tB_Phone.Text.Length == 0) { MessageBox.Show("姓名电话必须填写!"); return; } string sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888"; string sqlcom = "INSERT INTO Contact (Contact_Name,Contact_QQ,Contact_Phone,Contact_Email,Account_Username) VALUES(‘" + tB_Name.Text + "‘,‘" + tB_QQ.Text + "‘,‘" + tB_Phone.Text + "‘,‘" + tB_Email.Text + "‘,‘"+Login.txtLogin_Username+"‘)"; //执行添加操作 SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); int eq = com.ExecuteNonQuery(); if (eq != 0) { MessageBox.Show("添加成功!"); } con.Close(); //清空所有文本框 foreach (Control item in this.Controls) { if (item is TextBox) { item.Text = ""; } } } } }
修改密码:Modification_Password
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; namespace Contact_Manage { //修改密码 public partial class Modification_Password : Form { public Modification_Password() { InitializeComponent(); } //修改密码事件 private void btt_Modification_Password_Click(object sender, EventArgs e) { //获取当前登录用户的登录名 String modification_Username = Login.txtLogin_Username; //获取用户输入的登录密码以及两次新密码 String login_Pwd = tB_Modif_Password.Text; String modif_NewPwd1 = tB_Modif_NewPassword.Text; String modif_NewPwd2 = tB_Modif_NewPassword2.Text; //判断是否输入了原密码 if (login_Pwd == "") { MessageBox.Show("请输入密码!"); return; } //连接数据库验证原密码是否正确 String sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888;"; String sqlcom = "select * from Account where Account_Password=‘"+login_Pwd+"‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom,con); con.Open(); SqlDataReader reader= com.ExecuteReader(); if (!reader.Read()) { MessageBox.Show("原登录密码错误,请重新输入!"); return; } con.Close(); //判断用户是否输入了新密码 if (modif_NewPwd1 == "" || modif_NewPwd2 == "") { MessageBox.Show("请输入新密码!"); return; } //判断两次输入的新密码是否一致 if (!modif_NewPwd1.Equals(modif_NewPwd2)) { MessageBox.Show("你两次输入的新密码不一致,请重新输入!"); return; } //验证通过,进行修改密码操作 String sqlcom2 = "update Account set Account_Password=‘"+modif_NewPwd1+"‘ where Account_Username=‘"+modification_Username+"‘"; SqlConnection con2 = new SqlConnection(sqlcon); SqlCommand com2 = new SqlCommand(sqlcom2, con2); con2.Open(); int eq=com2.ExecuteNonQuery(); if (eq != 0) { MessageBox.Show("修改密码成功思密达!"); this.Close(); } else MessageBox.Show("修改密码失败思密达!"); con2.Close(); } //当点击这个文本框时,将用户名显示 private void tB_Modif_Password_MouseClick(object sender, MouseEventArgs e) { //获取当前登录用户的登录名 String modification_Username = Login.txtLogin_Username; tB_Modif_Username.Text = modification_Username; } //当点击这个文本框时,也将用户名显示 private void tB_Modif_Username_MouseClick(object sender, MouseEventArgs e) { //获取当前登录用户的登录名 String modification_Username = Login.txtLogin_Username; tB_Modif_Username.Text = modification_Username; } } }
更换头像:Change_Image
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; namespace Contact_Manage { public partial class Change_Image : Form { public Change_Image() { InitializeComponent(); } private void btt_Select_Image_Click(object sender, EventArgs e) { //自定义OpenFileDialog打开Windows文件对话框选择文件,并保存路径 OpenFileDialog f = new OpenFileDialog(); f.InitialDirectory = Application.StartupPath + @"\images"; f.Filter = "All files (*.*)|*.*|txt files (*.txt)|*.txt"; f.FilterIndex = 1; f.RestoreDirectory = true; if (f.ShowDialog() == DialogResult.OK) { Regist.imagePath = f.FileName.ToString(); //将更换的图片显示在注册页面 pictrB_Image.Image = Image.FromFile(Regist.imagePath); //将图片居中显示 pictrB_Image.SizeMode = PictureBoxSizeMode.StretchImage; } return; } private void btt_Change_Image_Click(object sender, EventArgs e) { if (pictrB_Image.Image != null) { string sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888"; String sqlcom2 = "update Account set Account_Image=‘" + Regist.imagePath + "‘ where Account_Username=‘" + Login.txtLogin_Username + "‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com2 = new SqlCommand(sqlcom2, con); con.Open(); int eq = com2.ExecuteNonQuery(); if (eq != 0) { MessageBox.Show("头像更换成功!"); this.Close(); } else MessageBox.Show("头像更换失败!"); con.Close(); } else MessageBox.Show("请选择图片!"); } } }
修改账户名:Mdfct_Acct_Username
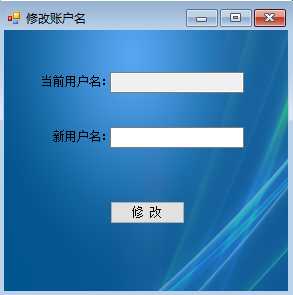
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; namespace Contact_Manage { public partial class Mdfct_Acct_Username : Form { public Mdfct_Acct_Username() { InitializeComponent(); } //点击此文本框时跳出当前登录名 private void tB_Username_MouseClick(object sender, MouseEventArgs e) { tB_Username.Text = Login.txtLogin_Username; } //点击此文本框时跳出当前登录名 private void tB_NewUsername_MouseClick(object sender, MouseEventArgs e) { tB_Username.Text = Login.txtLogin_Username; } private void btt_Modfct_Username_Click(object sender, EventArgs e) { //获取用户输入的新用户名 String newUsername = tB_NewUsername.Text; if (newUsername == "") { MessageBox.Show("请输入新用户名!"); return; } //连接数据库更改用户名 String sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888;"; String sqlcom = "update Account set Account_Username=‘"+newUsername+"‘ where Account_Username=‘"+Login.txtLogin_Username+"‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); int eq=com.ExecuteNonQuery(); if (eq != 0) { MessageBox.Show("修改成功!"); this.Close(); Login.txtLogin_Username = newUsername; } else MessageBox.Show("修改失败!"); } } }
联系人管理:Contact_Manage
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data.SqlClient; using System.Configuration; namespace Contact_Manage { public partial class Contact_Manage : Form { //数据库连接字符串 string sqlcon = "server=.;database=phone_Book;uid=sa;pwd=666888"; public Contact_Manage() { InitializeComponent(); } //查询事件 private void button1_Click(object sender, EventArgs e) { string condition = " 1=1"; //姓名 if (tB_Name.Text != "") condition = condition + " and Contact_Name like‘" + tB_Name.Text + "%‘"; //电话 if (tB_Phone.Text != "") condition = condition + " and Contact_Phone like‘" + tB_Phone.Text + "%‘"; //QQ if (tB_QQ.Text != "") condition = condition + " and Contact_QQ like‘" + tB_QQ.Text + "%‘"; //Email if (tB_Email.Text != "") condition = condition + " and Contact_Email like‘" + tB_Email.Text + "%‘"; //ID if (tB_ID.Text != "") { condition = condition + " and Contact_ID=‘" + tB_ID.Text + "‘"; } string sqlcom = "select * from Contact where" + condition; SqlConnection con = new SqlConnection(sqlcon); con.Open(); SqlDataAdapter adapter = new SqlDataAdapter(sqlcom, con); //设置默认列不显示 //dataGridView1.AutoGenerateColumns = false; DataSet ds = new DataSet(); adapter.Fill(ds,"cs"); dataGridView1.DataSource = ds.Tables["cs"];//绑定 con.Close(); //清空所有文本框 foreach (Control item in this.Controls) { if (item is TextBox) { item.Text = ""; } } //显示用户名 lb_Username.Text = Login.txtLogin_Username; String sqlcom2 = "select Account_Image from Account where Account_Username=‘" + Login.txtLogin_Username + "‘"; SqlConnection con2 = new SqlConnection(sqlcon); SqlCommand com2 = new SqlCommand(sqlcom2, con2); con2.Open(); //返回结果集的第一行第一列 object obj = com2.ExecuteScalar(); //将数据库中读取到的路径放入变量中 String image_Path = obj.ToString(); con2.Close(); pctrB_Image.Image = Image.FromFile(image_Path); //将图片居中显示 pctrB_Image.SizeMode = PictureBoxSizeMode.StretchImage; } //修改事件 private void btt_Modification_Click(object sender, EventArgs e) { //获取用户输入的要修改的联系人ID String modification_ID = tB_ID.Text; //如果文本框为空,则说明用户没有点击联系人 if (modification_ID != "") { String condition = ""; //姓名 /* if (tB_Name.Text != "") condition = condition + ",Contact_Name=‘" + tB_Name.Text + "‘";*/ //电话 if (tB_Phone.Text != "") condition = condition + ",Contact_Phone=‘" + tB_Phone.Text + "‘"; //QQ if (tB_QQ.Text != "") condition = condition + ",Contact_QQ=‘" + tB_QQ.Text + "‘"; //Email if (tB_Email.Text != "") condition = condition + ",Contact_Email=‘" + tB_Email.Text + "‘"; String sqlcom = "update Contact set Contact_Name=‘" + tB_Name.Text + "‘" + condition + " where Contact_ID = ‘" + modification_ID + "‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); int eq = com.ExecuteNonQuery(); if (eq != 0) { MessageBox.Show("修改成功思密达!"); } else MessageBox.Show("修改失败思密达!"); con.Close(); foreach (Control item in this.Controls) { if (item is TextBox) { item.Text = ""; } } } else MessageBox.Show("请先点击联系人再进行修改操作!"); } //删除事件 private void btt_Delete_Click(object sender, EventArgs e) { //获取用户输入的要删除的联系人ID String delete_ID = tB_ID.Text; //连接数据库将该联系人数据删除 String sqlcom = "delete from Contact where Contact_ID=‘" + delete_ID + "‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); int eq = com.ExecuteNonQuery(); if (eq != 0) { MessageBox.Show("删除成功思密达!"); } else MessageBox.Show("删除失败思密达!"); con.Close(); //清空文本框 foreach (Control item in this.Controls) { if (item is TextBox) { item.Text = ""; } } } //提示用户进行联系人管理时的操作说明 并显示用户名和头像 private void btt_Hint_Click(object sender, EventArgs e) { //显示用户名 lb_Username.Text = Login.txtLogin_Username; String sqlcom = "select Account_Image from Account where Account_Username=‘" + Login.txtLogin_Username + "‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); //返回结果集的第一行第一列 object obj = com.ExecuteScalar(); //将数据库中读取到的路径放入变量中 String image_Path = obj.ToString(); con.Close(); pctrB_Image.Image = Image.FromFile(image_Path); //将图片居中显示 pctrB_Image.SizeMode = PictureBoxSizeMode.StretchImage; MessageBox.Show("查询:文本框全为空时,点击查询将列出所有数据,除ID之外都为模糊查询。\r删除:点击要删除的行。\r修改:ID不可改"); } private void tB_Name_MouseClick(object sender, MouseEventArgs e) { //显示用户名 lb_Username.Text = Login.txtLogin_Username; String sqlcom = "select Account_Image from Account where Account_Username=‘" + Login.txtLogin_Username + "‘"; SqlConnection con = new SqlConnection(sqlcon); SqlCommand com = new SqlCommand(sqlcom, con); con.Open(); //返回结果集的第一行第一列 object obj = com.ExecuteScalar(); //将数据库中读取到的路径放入变量中 String image_Path = obj.ToString(); con.Close(); pctrB_Image.Image = Image.FromFile(image_Path); //将图片居中显示 pctrB_Image.SizeMode = PictureBoxSizeMode.StretchImage; } private void dataGridView1_CellMouseClick(object sender, DataGridViewCellMouseEventArgs e) { tB_ID.Text = this.dataGridView1.Rows[e.RowIndex].Cells[0].Value.ToString(); tB_Name.Text = this.dataGridView1.Rows[e.RowIndex].Cells[1].Value.ToString(); tB_QQ.Text = this.dataGridView1.Rows[e.RowIndex].Cells[2].Value.ToString(); tB_Phone.Text = this.dataGridView1.Rows[e.RowIndex].Cells[3].Value.ToString(); tB_Email.Text = this.dataGridView1.Rows[e.RowIndex].Cells[4].Value.ToString(); } } }
标签:
原文地址:http://www.cnblogs.com/xch-yang/p/5463676.html