package cn.sort;
/**
* @ClassName: Selection
* @Description: 选择排序类
* @author 无名
* @date 2016-6-9 下午7:23:59
* @version 1.0
*/
public class Selection extends Base
{
@Override
public void sort(Comparable[] arr)
{
int arrLength = arr.length;
for (int i = 0; i < arrLength; i++)
{
int min = i;
for(int j = i + 1; j < arrLength; j++)
{
if(less(arr[j], arr[min]))
{
min = j;
}
}
exch(arr, i, min);
}
}
}
AlgorithmTest.java:
package cn.test;
import org.junit.Test;
import cn.data.Date;
import cn.sort.Selection;
/**
* @ClassName: Test
* @Description: 测试类
* @author 无名
* @date 2016-6-9 下午7:26:08
* @version 1.0
*/
public class AlgorithmTest
{
private Date[] getTestData()
{
Date[] dateArr = {new Date(11,3,2001),new Date(11,7,1992),
new Date(10,12,1884),new Date(12,3,2001)};
return dateArr;
}
@Test
public void testSelection()
{
Selection selection = new Selection();
Date[] arr = getTestData();
selection.sort(arr);
selection.printArr(arr);
}
}
entity_class.h(C++没有java的toString()就只好自己写,输出时必须自己调用):
/*************************************************
Copyright:bupt
Author:无名
Date:2010-06-10
Description:实体类
**************************************************/
#ifndef ENTITY_H_
#define ENTITY_H_
#include <string>
using std::string;
class Date
{
private:
int day_;
int month_;
int year_;
public:
Date(int d, int m, int y);
string ToString();
inline bool operator< (const Date &date) const;
inline bool operator> (const Date &date) const;
inline bool operator== (const Date &date) const;
};
Date::Date(int d, int m, int y)
{
this->day_ = d;
this->month_ = m;
this->year_ = y;
}
string Date::ToString()
{
char ch_tmp_arr[10];
string final_str;
_itoa_s(this->year_, ch_tmp_arr, 10);
final_str.append(ch_tmp_arr);
final_str.append("-");
_itoa_s(this->month_, ch_tmp_arr, 10);
final_str.append(ch_tmp_arr);
final_str.append("-");
_itoa_s(this->day_, ch_tmp_arr, 10);
final_str.append(ch_tmp_arr);
return final_str;
}
inline bool Date::operator>(const Date &date) const
{
if (this->year_ > date.year_)
{
return true;
}
if (this->year_ < date.year_)
{
return false;
}
if (this->month_ > date.month_)
{
return true;
}
if (this->month_ < date.month_)
{
return false;
}
if (this->day_ > date.day_)
{
return true;
}
if (this->day_ < date.day_)
{
return false;
}
return 0;
}
inline bool Date::operator<(const Date &date) const
{
if (this->year_ > date.year_)
{
return false;
}
if (this->year_ < date.year_)
{
return true;
}
if (this->month_ > date.month_)
{
return false;
}
if (this->month_ < date.month_)
{
return true;
}
if (this->day_ > date.day_)
{
return false;
}
if (this->day_ < date.day_)
{
return true;
}
return 0;
}
inline bool Date::operator==(const Date &date) const
{
if (this->year_ == date.year_ && this->month_ == date.month_
&& this->day_ == date.day_)
return true;
return false;
}
#endif
sort_class.h(来看看C++坑爹的蠢乎乎的泛型吧):
/*************************************************
Copyright:bupt
Author:无名
Date:2010-06-10
Description:与排序相关的类
**************************************************/
#ifndef SORT_H_
#define SORT_H_
#include<iostream>
#include<string>
#include<vector>
using std::vector;
using std::cin;
using std::cout;
using std::endl;
template <class T>
class SortBase
{
public:
virtual void Sort(vector<T> & arr) = 0;
void Exch(vector<T> & arr, int i, int j);
void PrintArr(vector<T> & arr);
};
template <class T1, class T2>
class Selection :public SortBase<T1>
{
public:
void Sort(vector<T2> & arr);
};
template <class T>
void SortBase<T>::Exch(vector<T> & arr, int i, int j)
{
T t = arr[i];
arr[i] = arr[j];
arr[j] = t;
}
template <class T>
void SortBase<T>::PrintArr(vector<T> & arr)
{
int length = arr.size();
for (int i = 0; i < length - 1; i++)
{
cout << arr[i].ToString() << " ";
}
cout << arr[length - 1].ToString() << endl;
}
template <class T1, class T2>
void Selection<T1, T2>::Sort(vector<T2> & arr)
{
int arr_length = arr.size();
for (int i = 0; i < arr_length; i++)
{
int min = i;
for (int j = i + 1; j < arr_length; j++)
{
if (arr[j] < arr[min])
{
min = j;
}
}
Exch(arr, i, min);
}
}
#endif
algorithm_test.cpp:
/*************************************************
Copyright:bupt
Author:无名
Date:2010-06-10
Description:测试
**************************************************/
#include "sort_class.h"
#include "entity_class.h"
vector<Date> getTestData()
{
Date d1 = Date(11, 7, 1992);
Date d2 = Date(3, 2, 2001);
Date d3 = Date(10, 10, 1888);
Date d4 = Date(23, 7, 1992);
vector<Date> vec;
vec.push_back(d1);
vec.push_back(d2);
vec.push_back(d3);
vec.push_back(d4);
return vec;
}
void main()
{
vector<Date> vec = getTestData();
Selection<Date,Date> selection;
selection.Sort(vec);
selection.PrintArr(vec);
cin.get();
}
python实现(代码主要参考java,IDE eclipse,人生苦短,我用python你可以看到这些代码中,python的是最简洁的C++是最丑):
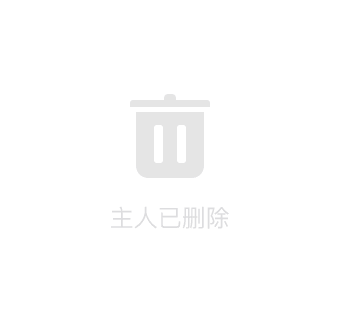
Date.py:
‘‘‘
Created on 2016-6-11
@author: sonn
‘‘‘
class Date:
def __init__(self,year,month,day):
self.__day = day
self.__month = month
self.__year = year
def compareTo(self,that):
if self.__year > that.__year:
return 1
if self.__year < that.__year:
return -1
if self.__month > that.__month:
return 1
if self.__month < that.__month:
return -1
if self.__day > that.__day:
return 1
if self.__day < that.__day:
return -1
return 0
def __repr__(self):
return str(self.__year) + "-" + str(self.__month) + "-" + str(self.__day)
Base.py:
‘‘‘
Created on 2016-6-11
@author: sonn
‘‘‘
from abc import ABCMeta, abstractmethod
class Base:
__metaclass__ = ABCMeta
@abstractmethod
def sort(self):pass
def less(self,v,w):
return v.compareTo(w)
def exch(self,arr,i,j):
tmp = arr[i]
arr[i] = arr[j]
arr[j] = tmp
def printArr(self,arr):
for date in arr:
print(date)
Selection.py:
‘‘‘
Created on 2016-6-11
@author: sonn
‘‘‘
from cn.sort.Base import Base
class Selection(Base):
def sort(self,arr):
arrLen = len(arr)
for i in range(0,arrLen-1):
min = i
for j in range(i + 1, arrLen - 1):
if(self.less(arr[j], arr[min]) < 0):
min = j
self.exch(arr, i, min)
Test.py:
‘‘‘
Created on 2016-6-11
@author: sonn
‘‘‘
from cn.data.Date import Date
from cn.sort.Selection import Selection
def getArrForSortTest():
date1 = Date(1992,7,11)
date2 = Date(2001,7,12)
date3 = Date(1777,6,13)
date4 = Date(2001,7,11)
arr = [date1,date2,date3,date4]
return arr
if __name__ == ‘__main__‘:
arr = getArrForSortTest()
selection = Selection()
selection.sort(arr)
selection.printArr(arr)