标签:utc inf 文本文件 set height 成功 文件流 位置 com
函数所在头文件:stdio.h
说明:前半部分主要为对各个文件流操作函数的例举,后半部分着重于
上机运行分析。文中部分引用自王桂林老师的C/C++课件。
1.FIELE *fopen(const char*filename,const char *mode)
以mode的方式,打开一个以filename(指针类型)命名的文件,
返回一个指向该文件缓冲区的指针,该指针是后续操作的句柄。
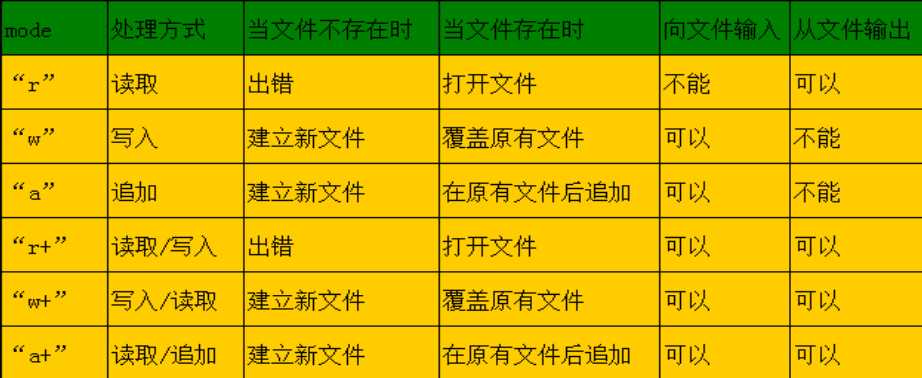
2.int fclose(FILE *stream)
fclose()用来关闭先前用fopen()打开的文件。并让文件缓冲
区的数据写入文件中,并释放系统提供的文件资源。成功范返回
0;失败返回-1(EOF)。
示例1:
#include <stdio.h>
int main()
{
FILE * fp = fopen("data.txt", "w");
//以"w"的方式打开一个名为"data.txt"的文件,文件不存在则创建
//判断文件是否都打开成功
if(fp == NULL)
{
printf("open error!\n");
return -1;
}
fputs("china is great!!", fp);
//向文件中写入"chia is great!!"
fclose(fp);
//关闭文件
return 0;
}
//该程序运行完毕,在该工程目录下生成了一个"data.txt"的文本文件,其内容为上述字符串
3.int fputc(int ch,FILE *stream)
将ch字符写入文件,成功返回写入字符,失败返回-1。
int fputs(char *str,FILE *fp)
将str指向的字符串写入fp指向的文件中,正常返回0;失败返回1.
示例2:
#include <stdio.h>
int main()
{
FILE* fp = fopen("data.txt","w");
if(fp == NULL)
{
printf("open error\n");
return -1;
}
char ch;
for(ch = ‘a‘; ch<=‘z‘; ch++)
printf("%3c",fputc(ch,fp));
//将字符写入文本的同时,将其打印出来
fclose(fp);
return 0;
}
程序运行结果:
同时,也在工程目录下生成了一个叫"data.txt"的文件,打开文件
可以发现其内容为a~z。
4.int fgetc(FILE *stream)
从文件流中读取一个字符并返回。成功返回读取的字符;读到文件
末尾或失败返回-1。
char *fgets(char *str,int length,FILE *fp)
从fp指向的文件中,至多读length-1个字符,送入数组str
中,如果在读入length-1个字符结束前遇到\n或EOF,读入即
结束,字符串读入后在最后加一个‘\0‘字符。
正常返回str指针,出错或遇到文件结尾,返回NULL指针。
示例3:
#include <stdio.h>
int main()
{
FILE* fp = fopen("data.txt","r");
//data.txt内容为a~z
if(fp == NULL)
{
printf("open error\n");
return -1;
}
char ch;
while((ch = fgetc(fp)) != EOF)
printf("%3c",ch);
fclose(fp);
return 0;
}
程序运行结果:
5.int feof(FILE *stream)
判断文件是否读到末尾,未读到末尾返回0,非零读到末尾。一般不
用,文件读到结尾,再去读一次,容易导致多读一次。不建议使用!
6.int fread(void* buffer,int num,int count,FILE *fp)
int fwrite(void*buffer,int num,int count,FILE *fp)
将buffer指向的数据写入fp指向的文件中,或是把fp指向的文件中的数
据读到buffer中,num为每个要读写的字段数的字节数,count为要读写
的字段数。成功返回读/写的字段数(count);出错或文件结束返回0。
这两个函数不同于其他函数,当我们试图用fread/fwrite去读写文本文
件的时候,发现文本中的格式己经没有任何意义,只是一个普通的字符。
它所进行的操作为二进制操作,通俗来说就是对一些文本标识符如‘\0‘,
‘\n‘等已经不敏感了,这些文本标识符都被当做成一个二进制来读写。
7.void rewind(FILE *STREAM)
将文件指针重新指向一个流的开头。
8.int ftell(FILE *stream)
得到流文件的当前读写位置,其返回值是当前读写位置偏离文件头
部的字节数。失败返回-1。
9.int fseek(FILE *stream,long offset,int where)
偏移文件指针,成功返回0,失败返回-1。where是偏移的起始位置。
//#define SEEK_CUR 1 当前位置
//#define SEEK_END 2 文件结尾
//#define SEEK_SET 0 文件开头
fseek(fp,100L,0);把fp指针移动到离文件开头100字节处;
fseek(fp,100L,1);把fp指针移动到离文件当前位置100字节处;
fseek(fp,-100,2);把fp指针退回到离文件结尾100字节处。
示例4:
#include <stdio.h>
int main()
{
FILE* fp = fopen("data.txt","w+");
if(fp == NULL)
{
printf("open error\n");
return -1;
}
char ch;
for( ch = ‘a‘; ch<=‘z‘; ch++)
{
fputc(ch,fp);
}
rewind(fp);
//此处一定不能少,否则文件指针将指向文件末尾,而打印不出任何东西
while((ch = fgetc(fp))&& !feof(fp))
{
printf("%3c",ch);
}
fclose(fp);
return 0;
}
程序运行结果:
示例5:
#include <stdio.h>
int main()
{
FILE* fp = fopen("data.txt","w+");
if(fp == NULL)
{
printf("open error\n");
return -1;
}
fputs("abcd\nefg",fp);
rewind(fp);
char ch;
while((ch = fgetc(fp)) != EOF)
printf("%c",ch);
fclose(fp);
return 0;
}
程序运行结果:
示例6:
下面是一个很有意思的程序,请判断下fgets()共执行了多少次;
先别看答案,相信这个理解了,这部分问题就不会太大,下面直接
上程序:
#include <stdio.h>
int main()
{
FILE* fp = fopen("data.txt","r");
if(fp == NULL)
{
printf("open error\n");
return -1;
}
char buf[10] = {0};
int count = 0;
while(fgets(buf,10,fp) != NULL) //注意,即使没遇到结束符,每次也只能读9个字符
{
printf("%s",buf);
count++;
}
fclose(fp);
putchar(10);
printf("+++++++++%d++++++++++",count);
return 0;
}
其中data.txt是这样的:
程序运行结果:
从运行结果来看,似乎是执行了5次,但仔细看看,真的是5次吗?
实际上,每读取一次,判断返回的指针是否为空,若不为空,则count加
1,若为空,则不执行循环体。因此,实际上是读取了6次,只是最后一
次读取后发现到了文件末尾,因此返回来一个空指针,判断为空指针
没执行count++操作。值得注意的是fgets()每次读取的字符数。
示例7:
#include <stdio.h>
int main()
{
FILE* fp = fopen("data.txt","w+");
if(fp == NULL)
{
printf("open error\n");
return -1;
}
fputs("china is great!!",fp);
int i = ftell(fp);
printf("%d\n",i);
//打印当前指针位置离文件头的字节数
rewind(fp);
//让文件指针指向文件头
i = ftell(fp);
printf("%d\n",i);
char buf[20] = {0};
fgets(buf,20,fp);
printf("%s\n",buf);
fclose(fp);
return 0;
}
程序运行结果:
示例8:
#include <stdio.h>
int main()
{
FILE* fp = fopen("data.txt","w+");
if(fp == NULL)
{
printf("open error\n");
return -1;
}
fputs("china is great!!",fp);
fseek(fp,2,0);
//将文件指针偏移到离文件头两个字节的位置
char buf[20];
fgets(buf,20,fp);
printf("%s\n",buf);
fclose(fp);
return 0;
}
程序运行结果:

示例9:
#include <stdio.h>
#include <string.h>
int main()
{
FILE *fpw = fopen("bin.txt","wb+");
if(fpw == NULL)
return -1;
char *p = "china \n is \0 great";
fwrite(p,1,strlen(p)+7,fpw);
//将指针的内容写入到文件中,由于strlen()所求长度
//遇到0截止,因此需将0后面的元素补上。
rewind(fpw);
char buf[1024];
int n = fread(buf,1,1024,fpw);
//每次读取一个字节,读取1024次,返回读取的字段数(此处为字节数)
//遇到文件末尾或读取错误返回0
printf("%d\n",n);
int i = 0;
for(i = 0;i<n;i++)
printf("%c",buf[i]);
fclose(fpw);
return 0;
}
程序运行结果:
标签:utc inf 文本文件 set height 成功 文件流 位置 com
原文地址:https://www.cnblogs.com/tuihou/p/9681160.html