标签:sch pack contains 服务器 criteria soft commit setimage setup
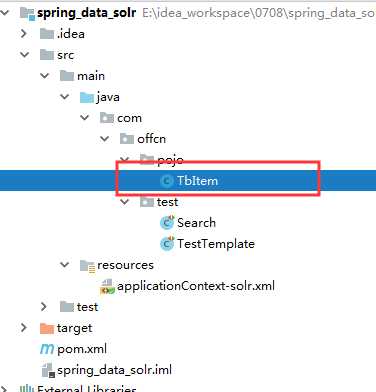
package com.offcn.pojo; import java.io.Serializable; import java.math.BigDecimal; import java.util.Date; import org.apache.solr.client.solrj.beans.Field; public class TbItem implements Serializable{ @Field private Long id; @Field("item_title") private String title; private String sellPoint; @Field("item_price") private BigDecimal price; private Integer stockCount; private Integer num; private String barcode; @Field("item_image") private String image; private Long categoryid; private String status; private Date createTime; private Date updateTime; private String itemSn; private BigDecimal costPirce; private BigDecimal marketPrice; private String isDefault; @Field("item_goodsid") private Long goodsId; private String sellerId; private String cartThumbnail; @Field("item_category") private String category; @Field("item_brand") private String brand; private String spec; @Field("item_seller") private String seller; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title == null ? null : title.trim(); } public String getSellPoint() { return sellPoint; } public void setSellPoint(String sellPoint) { this.sellPoint = sellPoint == null ? null : sellPoint.trim(); } public BigDecimal getPrice() { return price; } public void setPrice(BigDecimal price) { this.price = price; } public Integer getStockCount() { return stockCount; } public void setStockCount(Integer stockCount) { this.stockCount = stockCount; } public Integer getNum() { return num; } public void setNum(Integer num) { this.num = num; } public String getBarcode() { return barcode; } public void setBarcode(String barcode) { this.barcode = barcode == null ? null : barcode.trim(); } public String getImage() { return image; } public void setImage(String image) { this.image = image == null ? null : image.trim(); } public Long getCategoryid() { return categoryid; } public void setCategoryid(Long categoryid) { this.categoryid = categoryid; } public String getStatus() { return status; } public void setStatus(String status) { this.status = status == null ? null : status.trim(); } public Date getCreateTime() { return createTime; } public void setCreateTime(Date createTime) { this.createTime = createTime; } public Date getUpdateTime() { return updateTime; } public void setUpdateTime(Date updateTime) { this.updateTime = updateTime; } public String getItemSn() { return itemSn; } public void setItemSn(String itemSn) { this.itemSn = itemSn == null ? null : itemSn.trim(); } public BigDecimal getCostPirce() { return costPirce; } public void setCostPirce(BigDecimal costPirce) { this.costPirce = costPirce; } public BigDecimal getMarketPrice() { return marketPrice; } public void setMarketPrice(BigDecimal marketPrice) { this.marketPrice = marketPrice; } public String getIsDefault() { return isDefault; } public void setIsDefault(String isDefault) { this.isDefault = isDefault == null ? null : isDefault.trim(); } public Long getGoodsId() { return goodsId; } public void setGoodsId(Long goodsId) { this.goodsId = goodsId; } public String getSellerId() { return sellerId; } public void setSellerId(String sellerId) { this.sellerId = sellerId == null ? null : sellerId.trim(); } public String getCartThumbnail() { return cartThumbnail; } public void setCartThumbnail(String cartThumbnail) { this.cartThumbnail = cartThumbnail == null ? null : cartThumbnail.trim(); } public String getCategory() { return category; } public void setCategory(String category) { this.category = category == null ? null : category.trim(); } public String getBrand() { return brand; } public void setBrand(String brand) { this.brand = brand == null ? null : brand.trim(); } public String getSpec() { return spec; } public void setSpec(String spec) { this.spec = spec == null ? null : spec.trim(); } public String getSeller() { return seller; } public void setSeller(String seller) { this.seller = seller == null ? null : seller.trim(); } }
package com.offcn.test; import com.offcn.pojo.TbItem; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.solr.core.SolrTemplate; import org.springframework.data.solr.core.query.Criteria; import org.springframework.data.solr.core.query.Query; import org.springframework.data.solr.core.query.SimpleQuery; import org.springframework.data.solr.core.query.result.ScoredPage; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.math.BigDecimal; import java.util.ArrayList; import java.util.List; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext-solr.xml") public class TestTemplate { @Autowired private SolrTemplate solrTemplate; @Test public void test(){ TbItem item = new TbItem(); item.setId(1L); item.setBrand("华为"); item.setCategory("手机"); item.setGoodsId(1L); item.setSeller("华为2号专卖店"); item.setTitle("华为Mate9"); item.setPrice(new BigDecimal(2000)); solrTemplate.saveBean(item); solrTemplate.commit(); } @Test public void testFindOne(){ TbItem item = solrTemplate.getById(5, TbItem.class); System.out.println(item.getTitle()); } @Test public void testDelete(){ solrTemplate.deleteById("1"); solrTemplate.commit(); } @Test public void testAddList(){ List<TbItem> list=new ArrayList(); for(int i=0;i<100;i++){ TbItem item=new TbItem(); item.setId(i+1L); item.setBrand("华为"); item.setCategory("手机"); item.setGoodsId(1L); item.setSeller("华为2号专卖店"); item.setTitle("华为Mate"+i); item.setPrice(new BigDecimal(2000+i)); list.add(item); } solrTemplate.saveBeans(list); solrTemplate.commit(); } @Test public void testPage(){ Query query = new SimpleQuery("*:*"); query.setOffset(0);//开始索引(默认0) query.setRows(20);//每页记录数(默认10) ScoredPage<TbItem> page = solrTemplate.queryForPage(query, TbItem.class); System.out.println("总记录数:"+page.getTotalElements()); List<TbItem> list = page.getContent(); showList(list); } private void showList(List<TbItem> list) { for (TbItem item : list) { System.out.println(item.getTitle() ); } } @Test //分页查询 public void testQueryMutil(){ //创建查询对象 Query query = new SimpleQuery("*:*"); query.setOffset(0);//开始索引(默认0) query.setRows(20);//每页记录数(默认10) //声明一个查询条件 Criteria criteria = new Criteria("item_title").contains("5"); query.addCriteria(criteria); ScoredPage<TbItem> page = solrTemplate.queryForPage(query, TbItem.class); System.out.println("总记录数:"+page.getTotalElements()); List<TbItem> list = page.getContent(); showList(list); } @Test //删除所有 public void testDeleteAll(){ Query query = new SimpleQuery("*:*"); solrTemplate.delete(query); solrTemplate.commit(); } }
applicationContext-solr.xml配置:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:solr="http://www.springframework.org/schema/data/solr" xsi:schemaLocation="http://www.springframework.org/schema/data/solr http://www.springframework.org/schema/data/solr/spring-solr-1.0.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <!-- solr服务器地址 --> <solr:solr-server id="solrServer" url="http://192.168.200.128:8080/solr" /> <!-- solr模板,使用solr模板可对索引库进行CRUD的操作 --> <bean id="solrTemplate" class="org.springframework.data.solr.core.SolrTemplate"> <constructor-arg ref="solrServer" /> </bean> </beans>
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.offcn</groupId> <artifactId>spring_data_solr</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>org.springframework.data</groupId> <artifactId>spring-data-solr</artifactId> <version>1.5.5.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-test</artifactId> <version>4.2.4.RELEASE</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> </dependencies> </project>
标签:sch pack contains 服务器 criteria soft commit setimage setup
原文地址:https://www.cnblogs.com/chen4j/p/11908438.html