标签:ceo val ESS available mit json print auto options
MongoDB 创建数据库
语法
MongoDB 创建数据库的语法格式如下:
如果数据库不存在,则创建数据库,否则切换到指定数据库。
实例
以下实例我们创建了数据库 runoob:
> use runoob
switched to db runoob
> db
runoob
>
如果你想查看所有数据库,可以使用 show dbs 命令:
> show dbs
admin 0.000GB
config 0.000GB
local 0.000GB
>
可以看到,我们刚创建的数据库 runoob 并不在数据库的列表中, 要显示它,我们需要向 runoob 数据库插入一些数据。
> db.runoob.insert({"name":"菜鸟教程"})
WriteResult({ "nInserted" : 1 })
> show dbs
admin 0.000GB
config 0.000GB
local 0.000GB
runoob 0.000GB
MongoDB 中默认的数据库为 test,如果你没有创建新的数据库,集合将存放在 test 数据库中
MongoDB 删除数据库
语法
MongoDB 删除数据库的语法格式如下:
删除当前数据库,默认为 test,你可以使用 db 命令查看当前数据库名。
实例
以下实例我们删除了数据库 runoob。
首先,查看所有数据库:
> show dbs
admin 0.000GB
config 0.000GB
local 0.000GB
runoob 0.000GB
接下来我们切换到数据库 runoob:
> use runoob
switched to db runoob
>
执行删除命令:
> db.dropDatabase()
{ "dropped" : "runoob", "ok" : 1 }
最后,我们再通过 show dbs 命令数据库是否删除成功:
> show dbs
admin 0.000GB
config 0.000GB
local 0.000GB
删除集合
集合删除语法格式如下:
以下实例删除了 runoob 数据库中的集合 site:
> use runoob
switched to db runoob
> db.createCollection("runoob") # 先创建集合,类似数据库中的表
> show tables
runoob
> db.runoob.drop()
true
> show tables
>
MongoDB 创建集合
MongoDB 中使用 createCollection() 方法来创建集合。
语法格式:
db.createCollection(name, options)
参数说明:
- name: 要创建的集合名称
- options: 可选参数, 指定有关内存大小及索引的选项
options 可以是如下参数:
字段 | 类型 | 描述 |
capped |
布尔 |
(可选)如果为 true,则创建固定集合。固定集合是指有着固定大小的集合,当达到最大值时,它会自动覆盖最早的文档。 当该值为 true 时,必须指定 size 参数。 |
autoIndexId |
布尔 |
(可选)如为 true,自动在 _id 字段创建索引。默认为 false。 |
size |
数值 |
(可选)为固定集合指定一个最大值,以千字节计(KB)。 如果 capped 为 true,也需要指定该字段。 |
max |
数值 |
(可选)指定固定集合中包含文档的最大数量。 |
在插入文档时,MongoDB 首先检查固定集合的 size 字段,然后检查 max 字段。
实例
在 test 数据库中创建 runoob 集合:
> use test
switched to db test
> db.createCollection("runoob")
{ "ok" : 1 }
>
如果要查看已有集合,可以使用 show collections 或 show tables 命令:
> show collections
runoob
system.indexes
下面是带有几个关键参数的 createCollection() 的用法:
创建固定集合 mycol,整个集合空间大小 6142800 KB, 文档最大个数为 10000 个。
> db.createCollection("mycol", { capped : true, autoIndexId : true, size :
6142800, max : 10000 } )
{ "ok" : 1 }
>
在 MongoDB 中,你不需要创建集合。当你插入一些文档时,MongoDB 会自动创建集合。
> db.mycol2.insert({"name" : "测试"})
> show collections
mycol2
...
MongoDB 删除集合
本章节我们为大家介绍如何使用 MongoDB 来删除集合。
MongoDB 中使用 drop() 方法来删除集合。
语法格式:
参数说明:
返回值
如果成功删除选定集合,则 drop() 方法返回 true,否则返回 false。
实例
在数据库 mydb 中,我们可以先通过 show collections 命令查看已存在的集合:
>use mydb
switched to db mydb
>show collections
mycol
mycol2
system.indexes
runoob
>
接着删除集合 mycol2 :
通过 show collections 再次查看数据库 mydb 中的集合:
>show collections
mycol
system.indexes
runoob
>
从结果中可以看出 mycol2 集合已被删除。
MongoDB 插入文档
文档的数据结构和 JSON 基本一样。
所有存储在集合中的数据都是 BSON 格式。
BSON 是一种类似 JSON 的二进制形式的存储格式,是 Binary JSON 的简称。
Insert
插入单个
db.products.insert( { _id: 101, item: "box", qty: 20 } );
db.products.find();
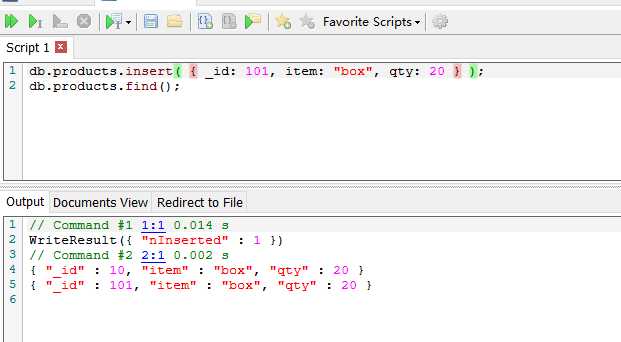
插入多个
db.products.insert(
[
{ _id: 11, item: "pencil", qty: 50, type: "no.2" },
{ item: "pen", qty: 20 },
{ item: "eraser", qty: 25 }
]
);
db.products.find();
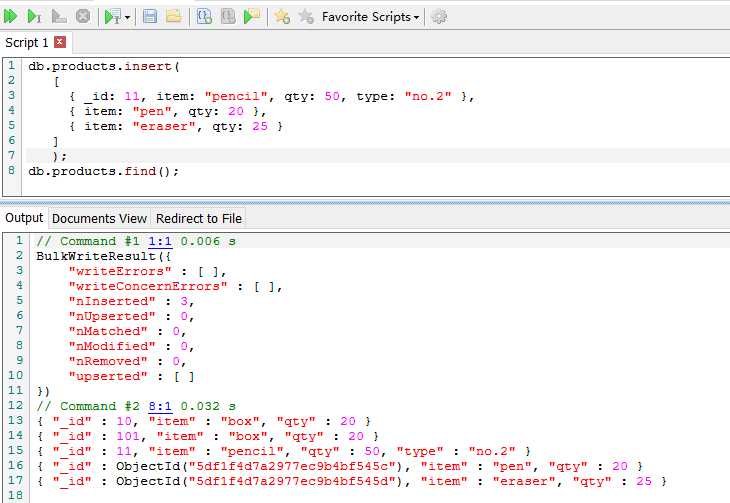
InsertOne
db.products.insertOne( { "item" : "packing peanuts", "qty" : 200 } );
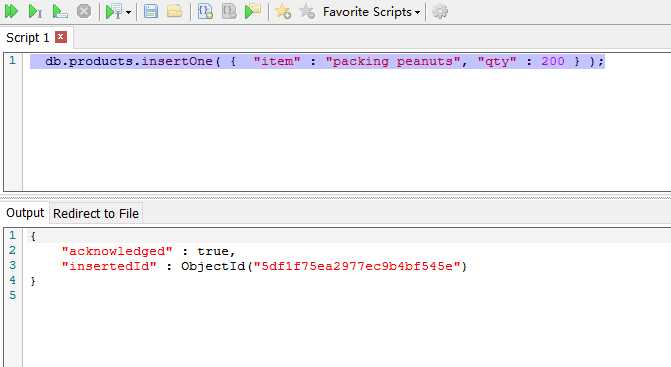
InsertMany
db.products.insertMany( [
{ item: "card", qty: 15 },
{ item: "envelope", qty: 20 },
{ item: "stamps" , qty: 30 }
] );
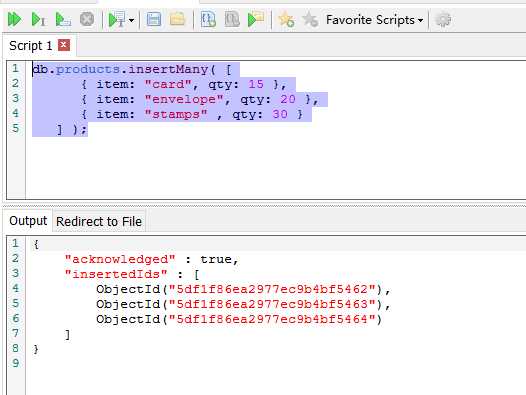
MongoDB 更新文档
MongoDB 使用 update() 和 save() 方法来更新集合中的文档。接下来让我们详细来看下两个函数的应用及其区别。
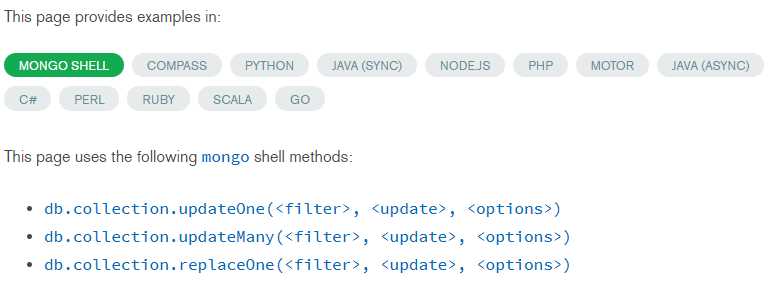
update() 方法
update() 方法用于更新已存在的文档。语法格式如下:
db.collection.update(
<query>,
<update>,
{
upsert: <boolean>,
multi: <boolean>,
writeConcern: <document>
}
)
具体查看:https://docs.mongodb.com/manual/reference/method/db.collection.update/
Parameter | Type | Description |
query |
document |
The selection criteria for the update. The same query selectors as in the find() method are available.
Changed in version 3.0: When you execute an update() with upsert: true and the query matches no existing document, MongoDB will refuse to insert a new document if the query specifies conditions on the _id field using dot notation.
|
update |
document or pipeline |
The modifications to apply. Can be one of the following:
Update document |
Contains only update operator expressions.
|
Replacement document |
Contains only <field1>: <value1> pairs.
|
Aggregation pipeline |
Consists only of the following aggregation stages:
$addFields and its alias $set
$project and its alias $unset
$replaceRoot and its alias $replaceWith .
|
For details and examples, see Examples.
|
upsert |
boolean |
Optional. If set to true , creates a new document when no document matches the query criteria. The default value is false , which does not insert a new document when no match is found.
|
multi |
boolean |
Optional. If set to true , updates multiple documents that meet the query criteria. If set to false , updates one document. The default value is false . For additional information, see Update Multiple Documents Examples.
|
writeConcern |
document |
Optional. A document expressing the write concern. Omit to use the default write concern w: 1 .
Do not explicitly set the write concern for the operation if run in a transaction. To use write concern with transactions, see Transactions and Write Concern.
For an example using writeConcern , see Override Default Write Concern.
|
collation |
document |
Optional.
Collation allows users to specify language-specific rules for string comparison, such as rules for lettercase and accent marks.
For an example using collation , see Specify Collation.
|
arrayFilters |
array |
Optional. An array of filter documents that determine which array elements to modify for an update operation on an array field.
In the update document, use the $[<identifier>] to define an identifier to update only those array elements that match the corresponding filter document in the arrayFilters .
NOTE
You cannot have an array filter document for an identifier if the identifier is not included in the update document.
For examples, see Specify arrayFilters for Array Update Operations.
|
hint |
Document or string |
Optional. A document or string that specifies the index to use to support the query predicate.
The option can take an index specification document or the index name string.
If you specify an index that does not exist, the operation errors.
For an example, see Specify hint for Update Operations.
|
参数说明:
- query : update的查询条件,类似sql update查询内where后面的。
- update : update的对象和一些更新的操作符(如$,$inc...)等,也可以理解为sql update查询内set后面的
- upsert : 可选,这个参数的意思是,如果不存在update的记录,是否插入objNew,true为插入,默认是false,不插入。
- multi : 可选,mongodb 默认是false,只更新找到的第一条记录,如果这个参数为true,就把按条件查出来多条记录全部更新。
- writeConcern :可选,抛出异常的级别。
实例
插入数据:
db.books.remove({});
db.books.insertMany([
{
"_id" : 1,
"item" : "TBD",
"stock" : 0,
"info" : { "publisher" : "1111", "pages" : 430 },
"tags" : [ "technology", "computer" ],
"ratings" : [ { "by" : "ijk", "rating" : 4 }, { "by" : "lmn", "rating" : 5 } ],
"reorder" : false
},
{
"_id" : 2,
"item" : "XYZ123",
"stock" : 15,
"info" : { "publisher" : "5555", "pages" : 150 },
"tags" : [ ],
"ratings" : [ { "by" : "xyz", "rating" : 5 } ],
"reorder" : false
}
]);
进行更新
db.books.update(
{ _id: 1 },
{
$inc: { stock: 5 },
$set: {
item: "ABC123",
"info.publisher": "2222",
tags: [ "software" ],
"ratings.1": { by: "xyz", rating: 3 }
}
}
)
类比sql
UPDATE books
SET stock = stock + 5
item = "ABC123"
publisher = 2222
pages = 430
tags = "software"
rating_authors = "ijk,xyz"
rating_values = "4,3"
WHERE _id = 1
upsert相当于oralce的merge,匹配到更新,不存在插入
db.books.update(
{ item: "ZZZ135" }, // Query parameter
{ // Replacement document
item: "ZZZ135",
stock: 5,
tags: [ "database" ]
},
{ upsert: true } // Options
)
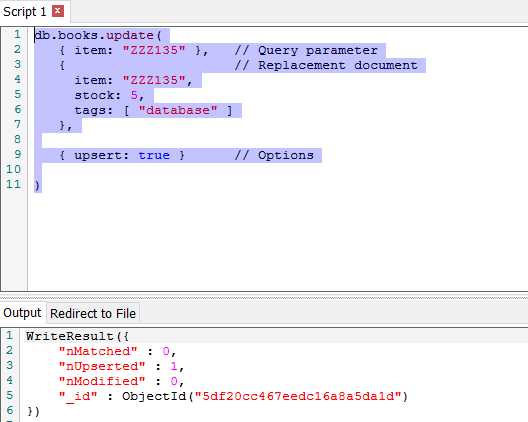
具体的参数含义,可以去官网查看
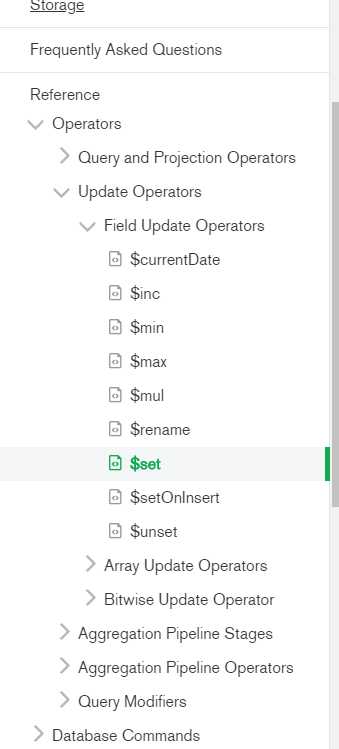
我们在集合中插入如下数据:
db.inventory.insertMany( [
{ item: "canvas", qty: 100, size: { h: 28, w: 35.5, uom: "cm" }, status: "A" },
{ item: "journal", qty: 25, size: { h: 14, w: 21, uom: "cm" }, status: "A" },
{ item: "mat", qty: 85, size: { h: 27.9, w: 35.5, uom: "cm" }, status: "A" },
{ item: "mousepad", qty: 25, size: { h: 19, w: 22.85, uom: "cm" }, status: "P" },
{ item: "notebook", qty: 50, size: { h: 8.5, w: 11, uom: "in" }, status: "P" },
{ item: "paper", qty: 100, size: { h: 8.5, w: 11, uom: "in" }, status: "D" },
{ item: "planner", qty: 75, size: { h: 22.85, w: 30, uom: "cm" }, status: "D" },
{ item: "postcard", qty: 45, size: { h: 10, w: 15.25, uom: "cm" }, status: "A" },
{ item: "sketchbook", qty: 80, size: { h: 14, w: 21, uom: "cm" }, status: "A" },
{ item: "sketch pad", qty: 95, size: { h: 22.85, w: 30.5, uom: "cm" }, status: "A" }
] );
接着我们通过 update() 方法来更新标题(title):
db.inventory.updateOne(
{ item: "paper" },
{
$set: { "size.uom": "cm", status: "P" },
$currentDate: { lastModified: true }
}
)
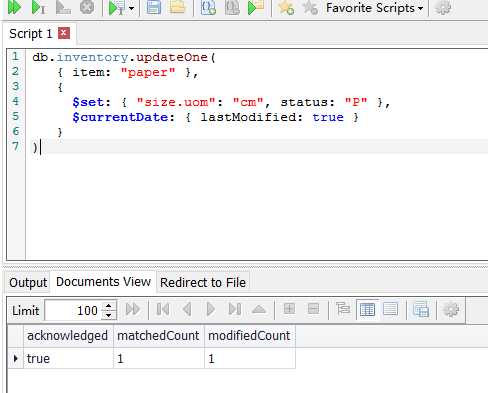
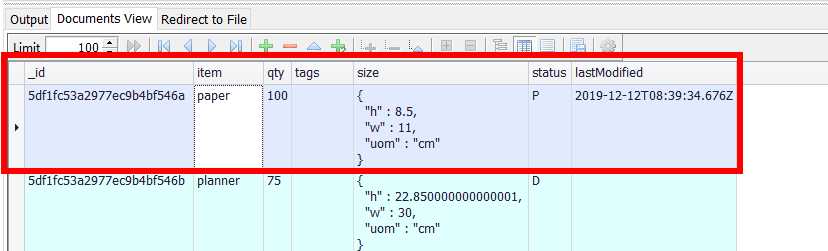
db.inventory.updateMany(
{ "qty": { $lt: 50 } },
{
$set: { "size.uom": "in", status: "P" },
$currentDate: { lastModified: true }
}
)
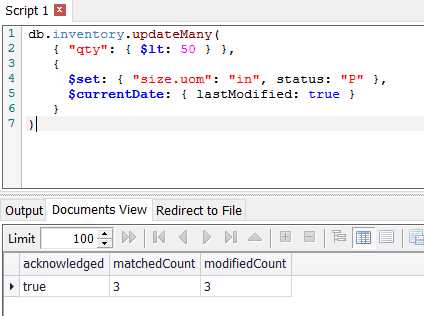
db.inventory.replaceOne(
{ item: "paper" },
{ item: "paper", instock: [ { warehouse: "A", qty: 60 }, { warehouse: "B", qty: 40 } ] }
)
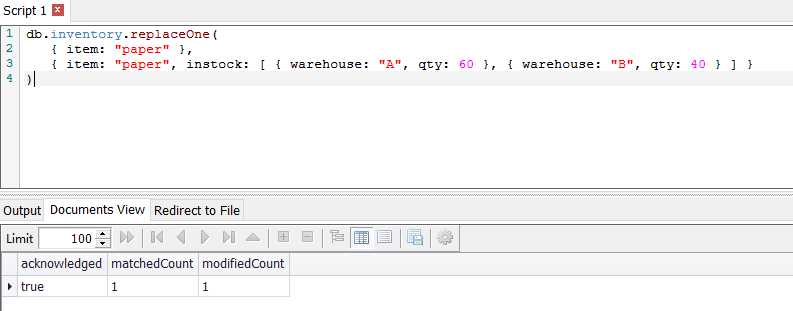
MongoDB 删除文档
MongoDB remove()函数是用来移除集合中的数据。
MongoDB数据更新可以使用update()函数。在执行remove()函数前先执行find()命令来判断执行的条件是否正确,这是一个比较好的习惯。
语法
remove() 方法的基本语法格式如下所示:
db.collection.remove(
<query>,
<justOne>
)
如果你的 MongoDB 是 2.6 版本以后的,语法格式如下:
db.collection.remove(
<query>,
{
justOne: <boolean>,
writeConcern: <document>
}
)
参数说明:
- query :(可选)删除的文档的条件。
- justOne : (可选)如果设为 true 或 1,则只删除一个文档,如果不设置该参数,或使用默认值 false,则删除所有匹配条件的文档。
- writeConcern :(可选)抛出异常的级别。
实例
db.products.remove( { qty: { $gt: 20 } } )
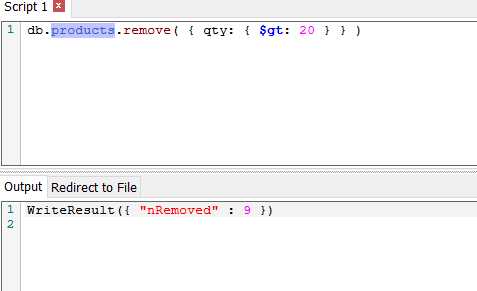
db.products.remove(
{ qty: { $lt: 20 } },
{ writeConcern: { item: "card", wtimeout: 5000 } }
)
MongoDB 查询文档
个人感觉查询的用法有点像MybatisPlus的语法
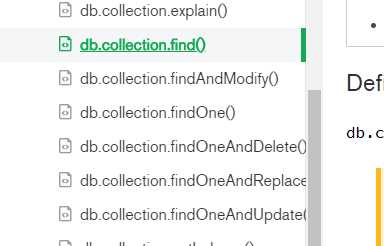
具体可以看一下官网的例子
MongoDB 查询文档使用 find() 方法。
find() 方法以非结构化的方式来显示所有文档。
语法
MongoDB 查询数据的语法格式如下:
db.collection.find(query, projection)
- query :可选,使用查询操作符指定查询条件
- projection :可选,使用投影操作符指定返回的键。查询时返回文档中所有键值, 只需省略该参数即可(默认省略)。
如果你需要以易读的方式来读取数据,可以使用 pretty() 方法,语法格式如下:
>db.col.find().pretty()
pretty() 方法以格式化的方式来显示所有文档。
实例
db.bios.find().sort( { name: 1 } ).limit( 5 )
db.bios.find().limit( 5 ).sort( { name: 1 } )
db.bios.find(
{ awards: { $elemMatch: { award: "Turing Award", year: { $gt: 1980 } } } }
)
db.bios.find( {
birth: { $gt: new Date(‘1920-01-01‘) },
death: { $exists: false }
} )
除了 find() 方法之外,还有一个 findOne() 方法,它只返回一个文档。
findOne条件
db.bios.findOne(
{
$or: [
{ ‘name.first‘ : /^G/ },
{ birth: { $lt: new Date(‘01/01/1945‘) } }
]
}
)
实例
db.bios.findOne(
{
$or: [
{ ‘name.first‘ : /^G/ },
{ birth: { $lt: new Date(‘01/01/1945‘) } }
]
}
)
findAndModify使用
db.collection.findAndModify({
query: <document>,
sort: <document>,
remove: <boolean>,
update: <document or aggregation pipeline>, // Changed in MongoDB 4.2
new: <boolean>,
fields: <document>,
upsert: <boolean>,
bypassDocumentValidation: <boolean>,
writeConcern: <document>,
collation: <document>,
arrayFilters: [ <filterdocument1>, ... ]
});
MongoDb的增删改查
标签:ceo val ESS available mit json print auto options
原文地址:https://www.cnblogs.com/dalianpai/p/12030426.html