标签:oca width tar aik 资料 only 异常 read 编辑
前言
之前unity5.x在代码中写了debug.log..等等,打包之后在当前程序文件夹下会有个对应的"outlog.txt",2017之后这个文件被移到C盘用户Appdata/LocalLow/公司名 文件夹下面。觉得不方便就自己写了个
代码
-
-
-
-
using System.Diagnostics;
-
using Debug = UnityEngine.Debug;
-
-
-
-
-
private FileStream fileStream;
-
private StreamWriter streamWriter;
-
-
private bool isEditorCreate = false;
-
private int showFrames = 1000;
-
-
-
private static readonly object obj = new object();
-
private static DebugTrace m_instance;
-
public static DebugTrace Instance
-
-
-
-
-
-
-
-
-
m_instance = new DebugTrace();
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
if (Debug.unityLogger.logEnabled)
-
-
if (Application.isEditor)
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
private void Application_logMessageReceivedThreaded(string logString, string stackTrace, LogType type)
-
-
-
if (type != LogType.Warning)
-
-
-
StackTrace stack = new StackTrace(true);
-
string stackStr = string.Empty;
-
-
int frameCount = stack.FrameCount;
-
if (this.showFrames > frameCount) this.showFrames = frameCount;
-
-
-
for (int i = stack.FrameCount - this.showFrames; i < stack.FrameCount; i++)
-
-
StackFrame sf = stack.GetFrame(i);
-
-
stackStr += "at [" + sf.GetMethod().DeclaringType.FullName +
-
"." + sf.GetMethod().Name +
-
".Line:" + sf.GetFileLineNumber() + "]\n ";
-
-
-
-
-
-
-
string content = string.Format("time: {0} logType: {1} logString: {2} \nstackTrace: {3} {4} ",
-
DateTime.Now.ToString("HH:mm:ss"), type, logString, stackStr, "\r\n");
-
streamWriter.WriteLine(content);
-
-
-
-
private void CreateOutlog()
-
-
if (!Directory.Exists(Application.dataPath + "/../" + "OutLog"))
-
Directory.CreateDirectory(Application.dataPath + "/../" + "OutLog");
-
string path = Application.dataPath + "/../OutLog" + "/" + DateTime.Now.ToString("yyyyMMddHHmmss") + "_log.txt";
-
fileStream = new FileStream(path, FileMode.OpenOrCreate, FileAccess.ReadWrite);
-
streamWriter = new StreamWriter(fileStream);
-
Application.logMessageReceivedThreaded += Application_logMessageReceivedThreaded;
-
-
-
-
-
-
-
-
Application.logMessageReceivedThreaded -= Application_logMessageReceivedThreaded;
-
-
-
-
-
-
-
-
-
-
-
-
-
public void SetLogOptions(bool logEnable, int showFrams = 1, LogType filterLogType = LogType.Log, bool editorCreate = false)
-
-
Debug.unityLogger.logEnabled = logEnable;
-
Debug.unityLogger.filterLogType = filterLogType;
-
isEditorCreate = editorCreate;
-
this.showFrames = showFrams == 0 ? 1000 : showFrams;
-
-
-
关于 filterLogType
filterLogType默认设置是Log,会显示所有类型的Log。
Warning:会显示Warning,Assert,Error,Exception
Assert:会显示Assert,Error,Exception
Error:显示Error和Exception
Exception:只会显示Exception
使用
-
-
-
public class Test : MonoBehaviour
-
-
private BoxCollider boxCollider;
-
-
-
DebugTrace.Instance.SetLogOptions(true, 2, editorCreate: true);
-
DebugTrace.Instance.StartTrace();
-
-
-
Debug.LogError("LogError");
-
Debug.LogAssertion("LogAssertion");
-
-
boxCollider.enabled = false;
-
-
-
private void OnApplicationQuit()
-
-
DebugTrace.Instance.CloseTrace();
-
-
如果在编辑器中也设置产生日志,日志文件在当前项目路径下,打包后在exe同级目录下
在打包发布后某些数据会获取不到 例如行号
StackFrame参考
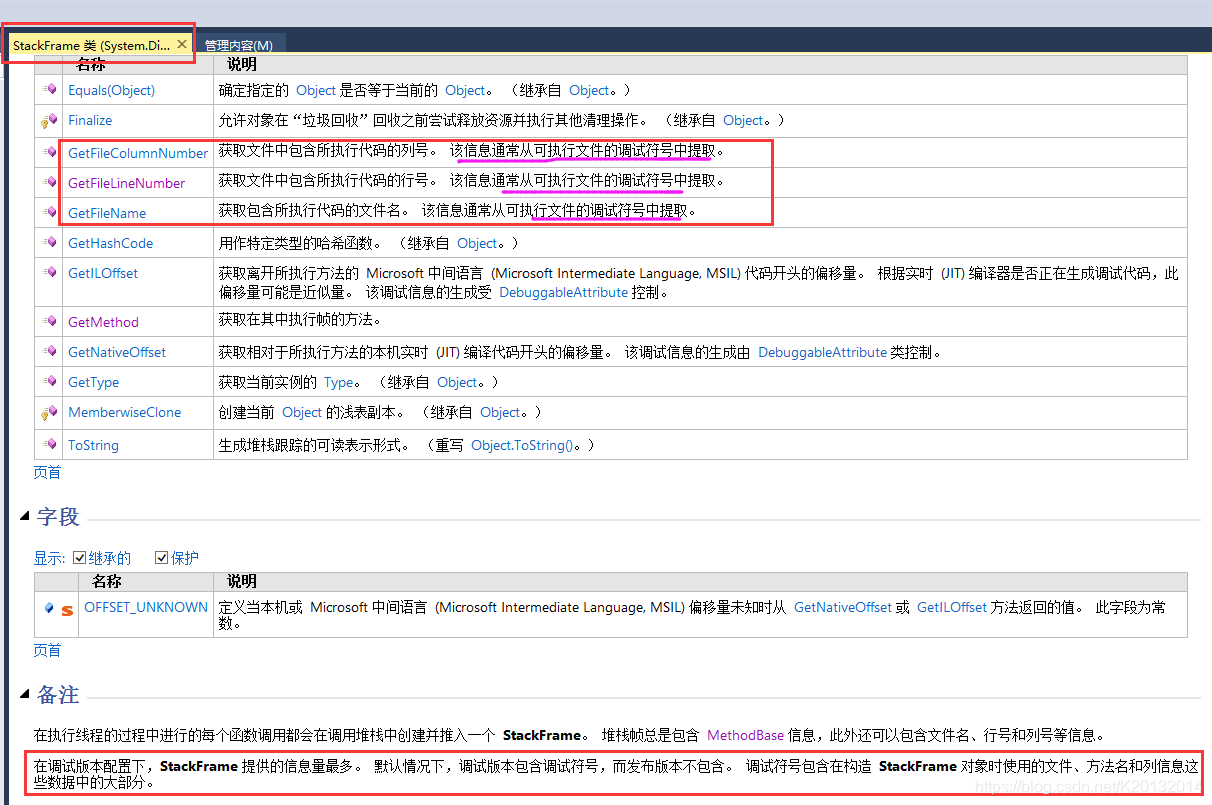
最后看下效果:
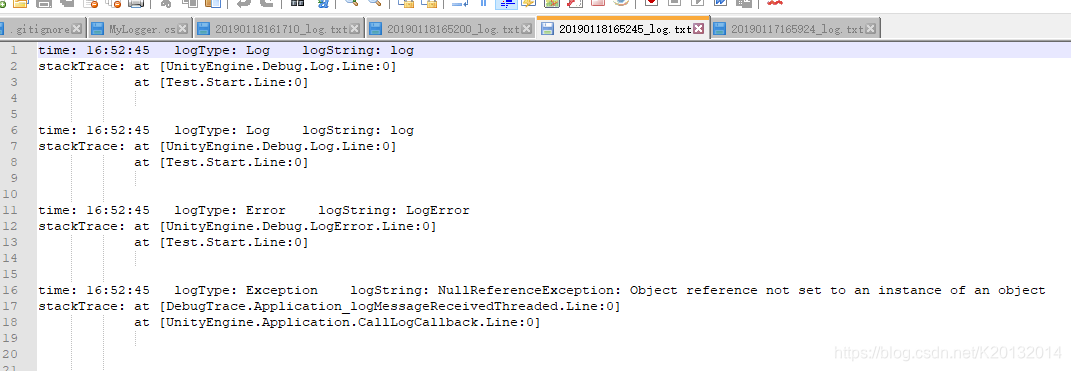
不足
发布版本 出现异常捕捉不到 行号获取不到
debug版本可以勾选DevelopMend build 捕捉到更多信息
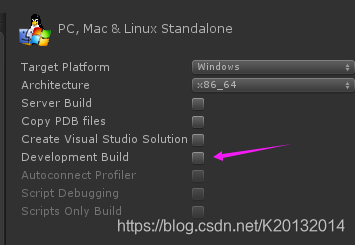
参考资料:
植物大战僵尸破解版: http://www.pvzbaike.com/archives/pvz_pojie/
Unity 自定义日志保存
标签:oca width tar aik 资料 only 异常 read 编辑
原文地址:https://www.cnblogs.com/geniushuai/p/14408737.html