标签:
程序代码
using System;
using System.IO;
using System.Net;
using System.Text;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
//构造soap请求信息
StringBuilder soap = new StringBuilder();
soap.Append("<?xml version=\"1.0\" encoding=\"utf-8\"?>");
soap.Append("<soap:Envelope xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\" xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\">");
soap.Append("<soap:Body>");
soap.Append("<getWeather xmlns=\"http://WebXml.com.cn/\">");
soap.Append("<theCityCode>2210</theCityCode>");
soap.Append("<theUserID></theUserID>");
soap.Append("</getWeather>");
soap.Append("</soap:Body>");
soap.Append("</soap:Envelope>");
//发起请求
Uri uri = new Uri("http://www.webxml.com.cn/WebServices/WeatherWS.asmx");
WebRequest webRequest = WebRequest.Create(uri);
webRequest.ContentType = "text/xml; charset=utf-8";
webRequest.Method = "POST";
using (Stream requestStream = webRequest.GetRequestStream())
{
byte[] paramBytes = Encoding.UTF8.GetBytes(soap.ToString());
requestStream.Write(paramBytes, 0, paramBytes.Length);
}
//响应
WebResponse webResponse = webRequest.GetResponse();
using (StreamReader myStreamReader = new StreamReader(webResponse.GetResponseStream(), Encoding.UTF8))
{
Console.WriteLine(myStreamReader.ReadToEnd());
}
Console.ReadKey();
}
}
}
返回结果:
引用内容
<?xml version="1.0" encoding="utf-8"?><soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"><soap:Body><getWeatherResponse xmlns="http://WebXml.com.cn/"><getWeatherResult><string>福建 福州</string><string>福州</string><string>2210</string><string>2010/01/21 15:42:15</string><string>今日天气实况:气温:12.5℃;风向/风力:东南风 4级;湿度:94%;气压:1011.9hPa</string><string>空气质量:良;紫外线强度:暂无</string><string>穿衣指数:建议着薄型套装或牛仔衫裤等春秋过渡装。年老体弱者宜着套装、夹克衫等。
感冒指数:将有一次强降温过程,且空气湿度较大,极易发生感冒,请特别注意增加衣服保暖防寒。
晨练指数:有阵雨,较不宜晨练,若坚持室外锻炼,请携带雨具。建议年老体弱人群适当减少晨练时间。
洗车指数:不宜洗车,未来24小时内有雨,如果在此期间洗车,雨水和路上的泥水可能会再次弄脏您的爱车。
晾晒指数:偶尔的降雨可能会淋湿晾晒的衣物,不太适宜晾晒。请随时注意天气变化。
旅游指数:有阵雨,温度适宜,在细雨中游玩别有一番情调,可不要错过机会呦!但记得出门要携带雨具。
路况指数:有小雨,路面潮湿,车辆易打滑,请小心驾驶。
舒适度指数:白天不太热也不太冷,风力不大,相信您在这样的天气条件下,应会感到比较清爽和舒适。</string><string>1月21日 阵雨转小雨</string><string>12℃/19℃</string><string>无持续风向微风</string><string>3.gif</string><string>7.gif</string><string>1月22日 小雨</string><string>9℃/14℃</string><string>无持续风向微风</string><string>7.gif</string><string>7.gif</string><string>1月23日 小雨</string><string>8℃/12℃</string><string>无持续风向微风</string><string>7.gif</string><string>7.gif</string><string>1月24日 阴</string><string>12℃/17℃</string><string>无持续风向微风</string><string>2.gif</string><string>2.gif</string><string>1月25日 多云</string><string>13℃/18℃</string><string>无持续风向微风</string><string>1.gif</string><string>1.gif</string></getWeatherResult></getWeatherResponse></soap:Body></soap:Envelope>
ASP版
<%
Dim xmlHttp,soap
soap = soap & "<?xml version=""1.0"" encoding=""utf-8""?>"
soap = soap & "<soap:Envelope xmlns:soap=""http://schemas.xmlsoap.org/soap/envelope/"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"">"
soap = soap & "<soap:Body>"
soap = soap & "<getWeather xmlns=""http://WebXml.com.cn/"">"
soap = soap & "<theCityCode>2210</theCityCode>"
soap = soap & "<theUserID></theUserID>"
soap = soap & "</getWeather>"
soap = soap & "</soap:Body>"
soap = soap & "</soap:Envelope>"
Set xmlHttp = CreateObject("MSXML2.XMLHTTP")
xmlHttp.Open "post","http://www.webxml.com.cn/WebServices/WeatherWS.asmx",false
xmlHttp.SetRequestHeader "Content-Type","text/xml; charset=utf-8"
‘曾见过Java的ws要求一定要有SOAPAction,否则报错no SOAPAction header!
xmlHttp.SetRequestHeader "SOAPAction","http://WebXml.com.cn/getWeather"
xmlHttp.Send(soap)
If xmlHttp.Status = 200 Then
Response.Write(xmlHttp.responseXML.xml)
End If
Set xmlHttp = Nothing
%>
带SoapHeader的Web Service调用
using System;
using System.IO;
using System.Net;
using System.Text;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
//构造soap请求信息
StringBuilder soap = new StringBuilder();
soap.Append("<?xml version=\"1.0\" encoding=\"utf-8\"?>");
soap.Append("<soap:Envelope xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\" xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\">");
soap.Append("<soap:Header>");
soap.Append("<MySoapHeader xmlns=\"http://www.mzwu.com/\" soap:mustUnderstand=\"1\">");//mustUnderstand=1,接收者必须能处理此SoapHeader信息,否则返回错误
soap.Append("<UserName>admin</UserName>");
soap.Append("<UserPass>admin888</UserPass>");
soap.Append("</MySoapHeader>");
soap.Append("</soap:Header>");
soap.Append("<soap:Body>");
soap.Append("<Hello xmlns=\"http://www.mzwu.com/\">");
soap.Append("<name>dnawo</name>");
soap.Append("</Hello>");
soap.Append("</soap:Body>");
soap.Append("</soap:Envelope>");
//发起请求
Uri uri = new Uri("http://localhost:39674/Service1.asmx");
WebRequest webRequest = WebRequest.Create(uri);
webRequest.ContentType = "text/xml; charset=utf-8";
webRequest.Method = "POST";
using (Stream requestStream = webRequest.GetRequestStream())
{
byte[] paramBytes = Encoding.UTF8.GetBytes(soap.ToString());
requestStream.Write(paramBytes, 0, paramBytes.Length);
}
//响应
WebResponse webResponse = webRequest.GetResponse();
using (StreamReader myStreamReader = new StreamReader(webResponse.GetResponseStream(), Encoding.UTF8))
{
Console.WriteLine(myStreamReader.ReadToEnd());
}
Console.ReadKey();
}
}
}
小技巧
在浏览器中查看Web Service时,有详细的SOAP请求和响应示例:
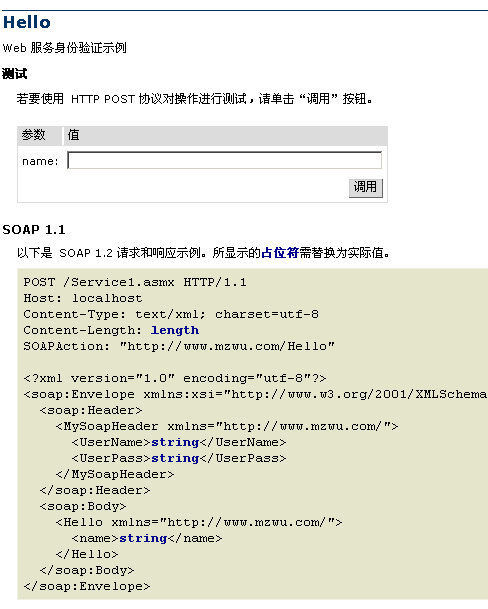
参考资料
·SOAP教程:http://www.w3school.com.cn/soap/index.asp
·简单对象访问协议:http://zh.wikipedia.org/zh-cn/SOAP
·SOAP调用Web Service:http://book.51cto.com/art/200906/129733.htm
·关于朗新WEBSERVER接口问题:http://fpcfjf.blog.163.com/blog/static/5546979320081069223827/
C#使用SOAP调用Web Service
标签:
原文地址:http://www.cnblogs.com/Alex80/p/4280871.html